An exception is an abnormal path of execution of code that the developer must handle.
Ok…then what is an error?
An error is an unexpected situation outside the control of the code that the developer should not try to handle.
An error may be caused due to environmental factors, hardware/virtual machine failures, etc.
None of these situations can be handed in our code and the developer should not even try to handle them in their code
Exception Objects
An Exception object is an object that will be created whenever an exception occurs.
There can be three types of constructors for creating exception objects.
- Exception(): An object of this kind is rarely observed as Java always tries to provide maximum information regarding the exception that occurred.
- Exception(String message): This kind of object provides an error message.
For Example: if our code is dividing a number by zero, then this object will say: / by 0 - Exception(String message, Throwable t): Throwable t gives us the stack trace i.e., the line number at which the exception occurs.
Note: Throwable is the superclass of all errors and exceptions.
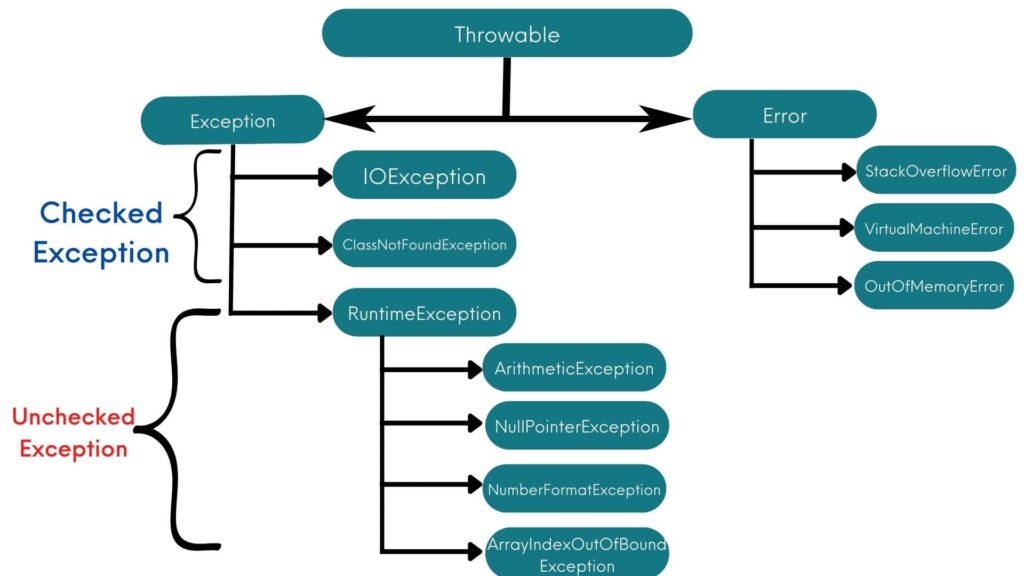
Note: The above flowchart presents the hierarchy for some important exceptions and errors, but there is a whole bunch of other errors and exceptions in Java that you will encounter as you move ahead in the journey of being a developer.
Checked/Unchecked Exception
Checked Exception: Checked Exceptions are exceptions that are resolved during compile time and hence if you have checked exceptions in your code, the code will not run at all.
All exceptions which are not descendants of Runtime Exception are checked exceptions.
Unchecked Exceptions: Unchecked Exceptions are exceptions that are resolved during runtime. All the exceptions that are descendants of Runtime Exception are unchecked exceptions.
User Defined Exceptions
Now, what happens if your business logic demands the handling of a situation that is not possible with the existing exceptions or if you want full control over when to introduce an exception??
In such cases, you can create custom exceptions.
Let’s see the syntax for defining custom exceptions.
/*Custom Exception Example*/
class MyCustomException extends Exception{
//code...
}
Note: If you extend Runtime Exception or any descendent of Runtime Exception, your custom exception will be unchecked exception else it will be checked exception.
Let’s wrap up…
In this blog, we have discussed Exceptions in Java. But as we know Java is vast and its vastness can sometimes be scary to new learners.
But not to worry as I’ll be covering most of the important topics that you can face while developing a website.
If your goal is to get a professional certification for Java you can refer to this course by Edureka and for an overall understanding of Java web development, you can refer to this course by Udacity.