In the previous blog, we understood what exceptions mean.
But how do we stop exceptions from crashing our application??
In this blog, we will find the answer to this question.
try-catch-finally
- try: This is where the problematic code goes. If you think a piece of your code can throw an exception, you must enclose it in the try block.
- catch: This is the code you want to execute when an exception has occurred while executing the try block. If the code in the try block doesn’t throw an exception, the catch block will not be run.
- finally: This is where the clean-up code goes. The finally block will run whether or not an exception occurs.
Let us see try-catch-finally in action.
try {
//the problematic piece of code
}
catch(ExceptionName e) {
//the code which should run when an exception with name "ExceptionName" occurs
}
finally {
//clean-up code
}
Note: Although finally block contains important clean-up code(like closing a database connection), it is optional.
There can be a possibility of more than one type of exception in a piece of code and hence there can be more than one catch block associated with a try block. But note that the exceptions handled in the catch block should always be in the order of most specific to least specific or else you will encounter code unreachable error (due to obvious reasons).
Also, if you have multiple catch blocks, the first catch block that can handle the exception will be run.
try {
//the problematic piece of code
}
catch(ArrayIndexOutOfBoundsException e) {
//the code which should run when an exception with
//name "ArrayIndexOutOfBoundsException" occurs
}
catch(IndexOutOfBoundsException e) {
//the code which should run when an exception with
//name "IndexOutOfBoundsException" occurs
}
catch(Exception e) {
//the code which should run when an exception with
//name "Exception" occurs
}
finally {
//clean-up code
}
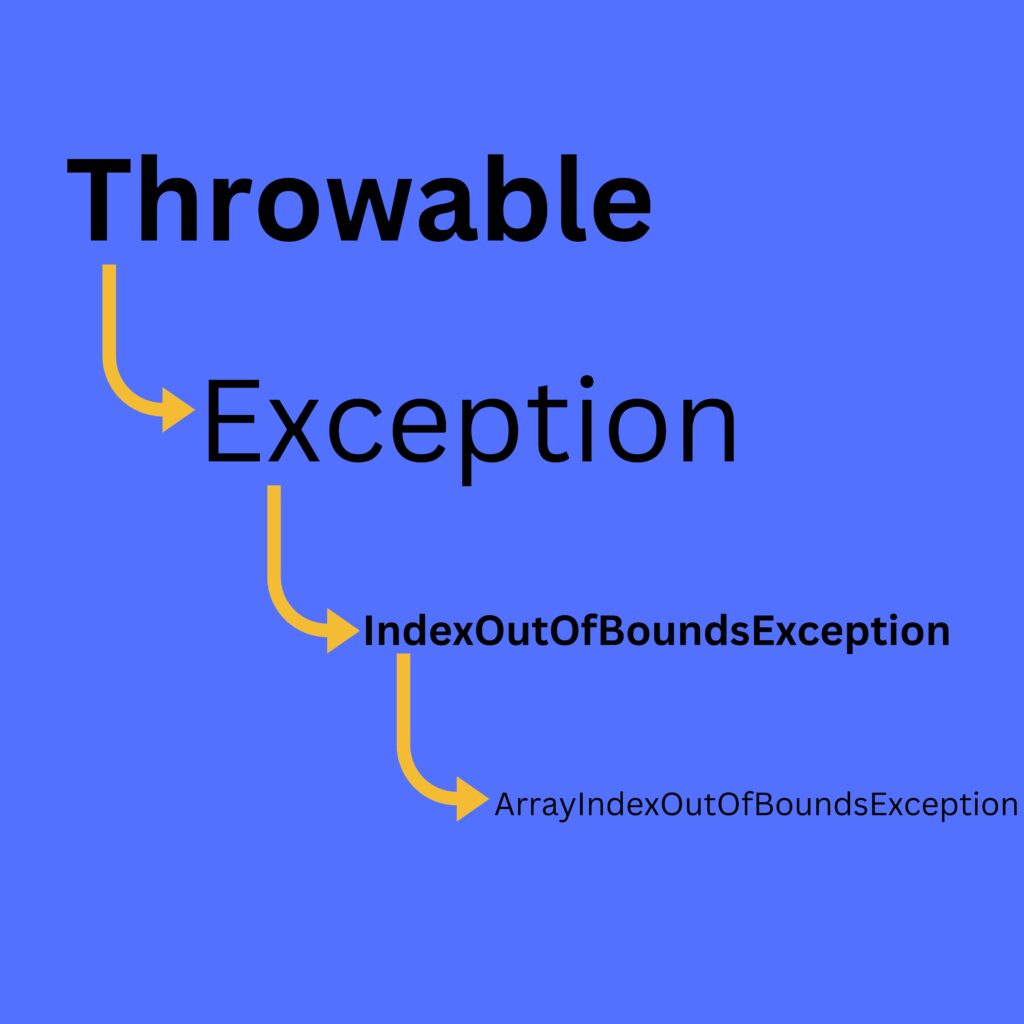
In newer versions of Java (after java 8), there is a smarter way to implement catch blocks if the body of the catch block remains the same but you need to handle multiple exceptions. All you need to do is separate the different exceptions in the catch block with a “|” symbol.
try {
//some code
}
catch(NullPointerException | ArrayIndexOutOfBoundsException | ArithmeticException e) {
System.err.println(e.getMessage());
}
Nested try-catch
If any inner try block does not have the catch for a particular exception, then the catch block of the outer exception attempts to catch the exception. If the outer catch block is also unable to catch the exception, its outer catch block tries to catch the exception, and so on. If none of the catch blocks are able to catch the exception, then it displays the system-generated message for that exception.
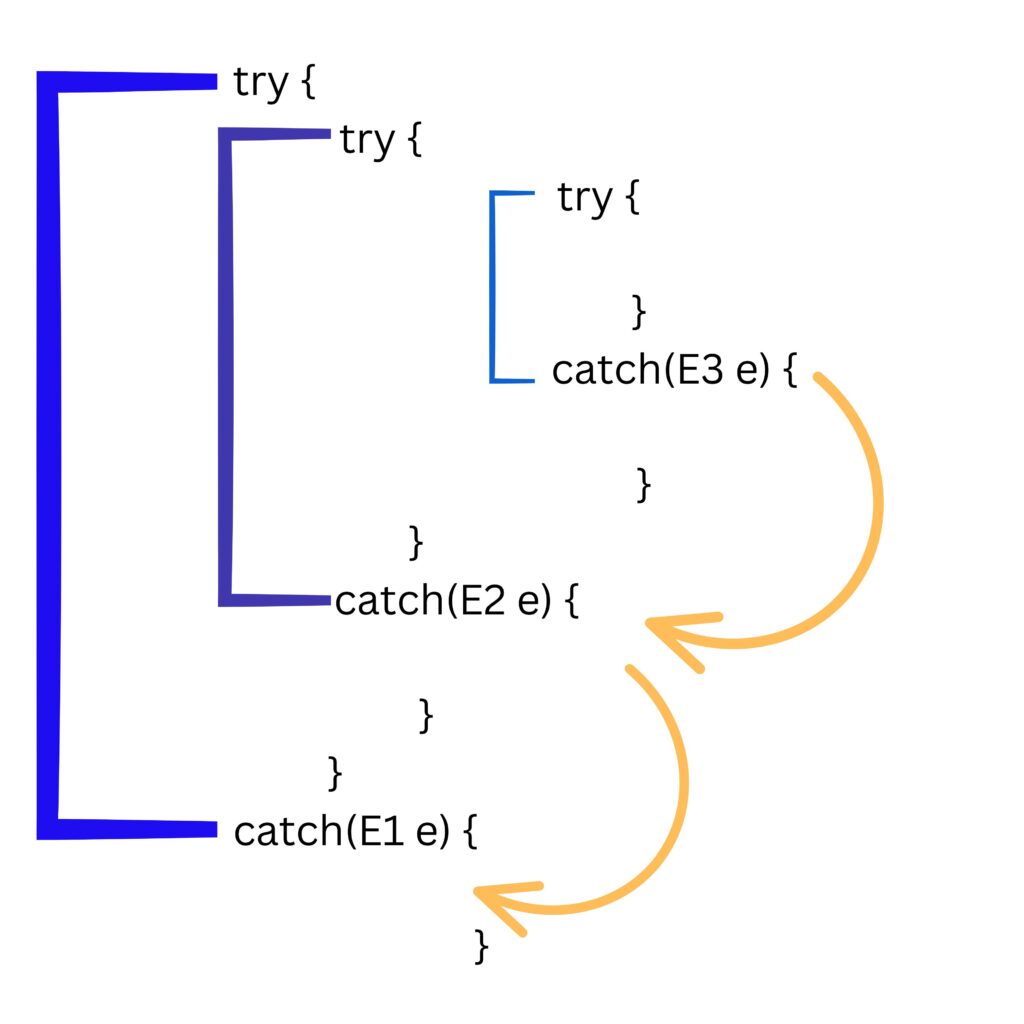
throw keyword
The throw keyword is used for throwing exceptions explicitly.
It is mainly used when you want to throw our own custom exceptions.
try {
throw new MyException();//MyException is a custom exceptions
}
catch(MyException e) {
//handle the exception
}
throws keyword
The throws keyword passes the responsibility of handling the exception from the callee method to the caller method.
See the below example for a clear understanding.
int method1(int x,int y) throws ArithmeticException {
//callee method
return x/y;
}
void method2() {
//caller method
try {
method1(10,0);// 10 divided by 0 generates ArithmeticException
}
catch(ArithmeticException e) {
System.err.println(e);
}
try-with-resources
The try-catch-finally is too verbose and if you forget to release resources in finally, it will continue to use memory which might prove costly!!!
The try-with-resources passes the responsibility of releasing resources to Java hence removing the need for finally block.
try (FileReader fr = new FileReader(filepath);BufferedReader br = new BufferedReader(bfr)) {
//business logic
}
catch(IOException e) {
//handle exception
}
Summing up…
In this blog, we have discussed Exception Handling in Java. But as we know Java is vast and its vastness can sometimes be scary to new learners.
But not to worry as I’ll be covering most of the important topics that you can face while developing a website.
If your goal is to get a professional certification for Java you can refer to this course by Edureka and for an overall understanding of Java web development, you can refer to this course by Udacity.