Polymorphism is a feature in OOP by which an entity can exhibit itself in multiple forms.
In Java, polymorphism can be achieved in the following ways
- Run-time polymorphism
- Compile-time polymorphism
Now let’s understand what each of these means.
Run-time Polymorphism
Run-time polymorphism is resolved by JVM(Java Virtual Machine) during execution and hence the name run-time polymorphism. Run-time polymorphism is achieved by method overriding.
If a child class provides an implementation of a method that has already been declared in one of its parent classes, it is known as method overriding.
So…what are the rules for method overriding??
Method overriding has the below three rules
- There must be an IS-A relationship(parent-child relationship)
- The method in the child class must have the same name as the method in the parent class
- The method in the child class must have the same parameters as the method in the parent class
/*Example of method overriding*/
class ParentClass{
public void method1(){
//method implementation of parent
//class
}
}
class BaseClass extends ParentClass{
@Override
public void method1(){
//method implementation of child
//class
}
}
public class Polymorphism {
public static void main(String[] args) {
BaseClass obj1=new BaseClass();//object of
//child class
obj1.method1();
}
}
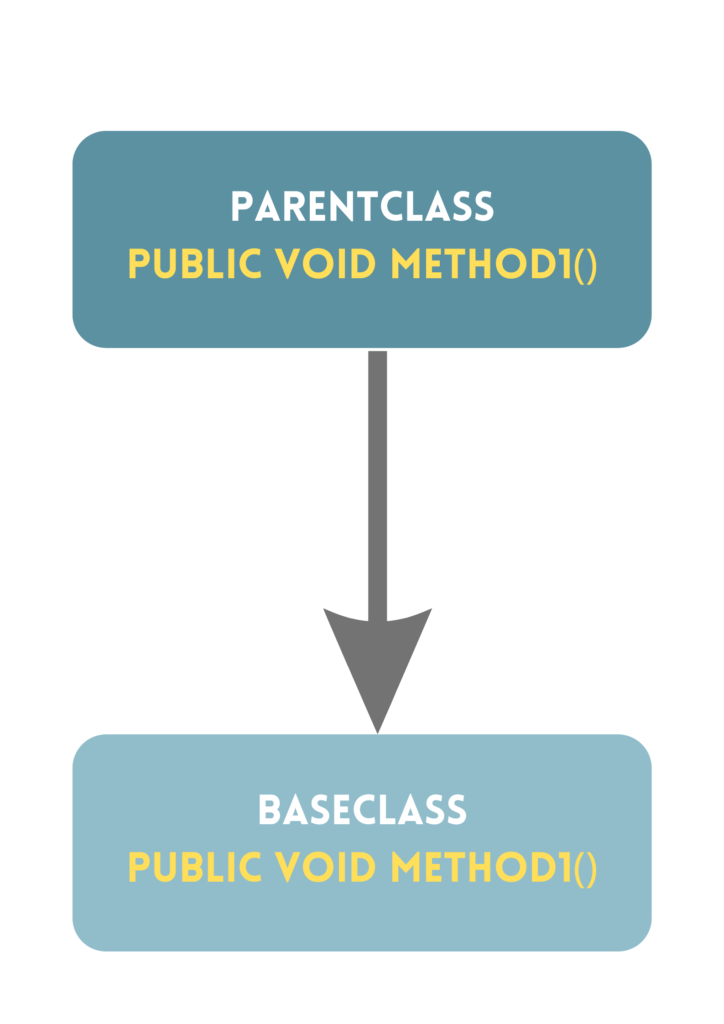
Compile-time Polymorphism
Unlike run-time polymorphism which is resolved by JVM(Java Virtual Machine), compile-time polymorphism is resolved by the compiler. Compile-time polymorphism is achieved by method overloading.
Method Overloading means that a given class will have more than one method with the same name but with different signatures.
/*Example of method overloading*/
class OverloadedClass{
public void method1(){ //overloaded method 1
//method1 with no parameters
}
public void method1(String str){//overloaded method 2
//method1 with a String parameter
}
public void method1(int a){//overloaded method 3
//method1 with an int parameter
}
public void method1(int a,int b){//overloaded method 4
//method1 with 2 int parameters
}
}
public class Polymorphism {
public static void main(String[] args) {
OverloadedClass obj1=new OverloadedClass();//object of OverloadedClass
obj1.method1();//overloaded method 1
obj1.method1("hello");//overloaded method 2
obj1.method1(4);//overloaded method 3
obj1.method1(5,6);//overloaded method 4
}
}
Summing it up…
In this blog, we have discussed Polymorphism in Java which is one of the most important principles of Object Oriented Programming. But as we know Java is vast and its vastness can sometimes be scary to new learners.
But not to worry as I’ll be covering most of the important topics that you can face while developing a website.
If your goal is to get a professional certification for Java you can refer to this course by Edureka and for an overall understanding of Java web development, you can refer to this course by Udacity.